<!DOCTYPE html>
Extensibility: Building Software That Adapts
<br> body {<br> font-family: sans-serif;<br> }</p> <div class="highlight"><pre class="highlight plaintext"><code>h1, h2, h3 { margin-top: 30px; } img { max-width: 100%; height: auto; } pre { background-color: #f0f0f0; padding: 10px; border-radius: 5px; overflow-x: auto; } code { font-family: monospace; } </code></pre></div> <p>
Extensibility: Building Software That Adapts
In the dynamic world of software development, adaptability is paramount. As requirements evolve, businesses need their software to keep pace. This is where extensibility comes into play. Extensible software, by design, allows for easy modification, expansion, and customization without requiring significant rework of the core codebase. In this comprehensive article, we'll delve into the concept of extensibility, exploring its significance, fundamental principles, and practical implementations.
Why Extensibility Matters
Extensibility offers numerous benefits, making it a crucial factor in building successful software applications:
-
Flexibility and Adaptability:
Extensible software can easily accommodate changing requirements, evolving business needs, and new features. It avoids rigid structures that hinder adaptation. -
Reduced Development Time and Costs:
By providing well-defined extension points, extensibility allows developers to focus on core functionality, while external developers or teams can contribute extensions without impacting the core codebase. This minimizes development time and costs. -
Increased Maintainability:
Well-structured extensions are easier to manage and maintain, making the software less prone to errors and facilitating future updates. -
Enhanced Reusability:
Reusable components and modules contribute to modularity, allowing for easier reuse of functionalities in different projects, saving time and effort. -
Community Engagement:
Open-source projects often leverage extensibility, enabling community contributions and fostering a vibrant ecosystem of plugins and extensions.

Key Concepts and Techniques
Extensibility is not a singular technique but a combination of principles and approaches that promote adaptability. Let's explore some of the key concepts and techniques used in building extensible software:
- Design Patterns
Design patterns are reusable solutions to common software design problems. Several patterns are particularly useful for promoting extensibility:
- Strategy Pattern: Defines a family of algorithms, encapsulating each one and making them interchangeable. This enables the software to adapt to different strategies without modifying the core code.
- Observer Pattern: Defines a one-to-many dependency between objects, where a change in one object triggers updates in its dependents. This allows for easy addition or removal of observers, making the software more flexible.
- Decorator Pattern: Dynamically adds responsibilities to an object. This allows for extending the functionality of existing objects without modifying their core code.
- Template Method Pattern: Defines the skeleton of an algorithm in a method, deferring some steps to subclasses. This allows subclasses to override specific steps, adapting the algorithm to their needs.
Interfaces and abstract classes define contracts that extensions must adhere to. This ensures that extensions are compatible with the core system and can be easily integrated.
// Interface example
interface IPaymentProcessor {
void processPayment(double amount);
}
// Concrete implementations
class PayPalProcessor implements IPaymentProcessor {
// ...
}
class StripeProcessor implements IPaymentProcessor {
// ...
}
- Plugin Architecture
A plugin architecture is a modular design where external components can be dynamically loaded and unloaded without requiring recompilation of the main application. This allows for a highly customizable and adaptable software.
Plugins typically follow these principles:
- Well-defined APIs: Plugins interact with the core system through clearly defined interfaces or APIs.
- Discovery mechanisms: The core system has a mechanism to discover and load available plugins.
- Configuration options: Plugins can be configured through settings files or other mechanisms to customize their behavior.
- Event-driven communication: Plugins can subscribe to events triggered by the core system and react accordingly.
Configuration files allow users to customize software behavior without modifying source code. This can include settings like database connections, API keys, and UI preferences.
# Example configuration file
database:
host: localhost
port: 3306
user: root
password: myPassword
An event-driven architecture allows components to communicate through events. Extensions can subscribe to specific events and react accordingly, adding new functionality or modifying existing behavior.
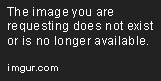
Advanced techniques like reflection and dynamic code generation allow for runtime introspection and code modification, enabling highly dynamic and flexible applications. However, these techniques should be used cautiously due to their complexity and potential performance implications.
Practical Examples and Tutorials
Let's illustrate the concept of extensibility with practical examples and tutorials:
Let's create a basic plugin system in Python using the entry_points mechanism provided by the setuptools package.
# core.py
import importlib
import pkg_resources
def load_plugins():
plugins = []
for entry_point in pkg_resources.iter_entry_points('my_plugins'):
plugin = entry_point.load()
plugins.append(plugin)
return plugins
if name == 'main':
plugins = load_plugins()
for plugin in plugins:
plugin.do_something()
plugin1.py
from my_plugin_interface import IPlugin
class Plugin1(IPlugin):
def do_something(self):
print("This is Plugin 1.")
plugin2.py
from my_plugin_interface import IPlugin
class Plugin2(IPlugin):
def do_something(self):
print("This is Plugin 2.")
setup.py:
from setuptools import setup
setup(
name='my_app',
version='0.1',
entry_points={
'my_plugins': [
'plugin1 = plugin1:Plugin1',
'plugin2 = plugin2:Plugin2',
]
}
)
In this example, we define a simple interface "IPlugin" and two concrete plugins that implement it. The core code dynamically loads available plugins using the "entry_points" mechanism and invokes their functionality.
- Extending a Web Application with Custom Filters
Let's extend a web application using custom filters in a framework like Django.
# my_app/filters.py
from django.http import HttpResponse
class MyFilter:
def process_request(self, request):
# Modify the request here (e.g., add headers)
return request
def process_response(self, request, response):
# Modify the response here (e.g., add custom content)
return response
my_app/urls.py
from django.urls import path
from django.views.generic import TemplateView
from .filters import MyFilter
urlpatterns = [
path('', TemplateView.as_view(template_name='index.html'), name='home'),
]
my_app/settings.py
MIDDLEWARE = [
# ... other middleware
'my_app.filters.MyFilter',
]
In this example, we create a custom filter "MyFilter" that can modify incoming requests and outgoing responses. The filter is registered in the "MIDDLEWARE" setting, allowing it to be executed for every request. This allows for customizing the application's behavior without modifying core views.
Conclusion
Extensibility is a powerful design principle that empowers software to adapt to evolving requirements and maintain its relevance in the long term. By employing key concepts like design patterns, interfaces, plugin architectures, and configuration files, developers can build software that is not only functional but also adaptable and future-proof.
When considering extensibility, it's essential to remember that:
-
Planning is crucial:
Consider potential future needs and design the system with extensibility in mind from the start. -
Balance is key:
While extensibility is beneficial, it should not come at the cost of complexity or performance. -
Documentation is vital:
Ensure clear documentation for developers to understand how to extend the system and create compatible components.
As the software landscape continues to evolve, extensibility will remain a vital factor in building applications that are adaptable, maintainable, and capable of meeting the ever-changing needs of users and businesses.