<!DOCTYPE html>
Methods vs Computed in Vue.js
<br> body {<br> font-family: sans-serif;<br> margin: 20px;<br> }<br> h1, h2, h3 {<br> color: #333;<br> }<br> code {<br> background-color: #eee;<br> padding: 5px;<br> border-radius: 3px;<br> }<br> img {<br> max-width: 100%;<br> display: block;<br> margin: 20px auto;<br> }<br>
Methods vs Computed Properties in Vue.js
Vue.js is a progressive JavaScript framework known for its simplicity and reactivity. One of the core concepts in Vue is its reactive system, which ensures that changes in data automatically update the user interface. Within this reactive system, two key mechanisms for managing data and UI updates are Methods and Computed Properties.
Introducing Vue.js and Its Reactivity System
Vue.js leverages a powerful reactivity system to streamline data updates and UI interactions. Here's how it works:
-
Data Binding:
Vue uses data binding to connect your data to the HTML template. This means when data changes, the corresponding HTML elements update automatically. -
Reactivity:
Vue tracks changes to your data. Any time a data property is modified, the framework automatically re-renders the affected parts of the template, ensuring the UI stays in sync. -
Dependency Tracking:
Vue intelligently keeps track of which parts of the template depend on which data properties. This means only the necessary parts of the UI are re-rendered when data changes.
This reactive system is what makes Vue.js so efficient and easy to work with. Let's explore how Methods and Computed Properties play a crucial role in this system.
Methods and Computed Properties: An Overview
Methods
Methods are functions defined within a Vue component. They allow you to perform actions or operations on your data. They can modify data, make API calls, or perform any other logic.
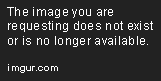
Here's a simple example:
<template>
<div>
<h1>
Counter: {{ counter }}
</h1>
<button @click="increment">
Increment
</button>
</div>
</template>
<script>
export default {
data() {
return {
counter: 0
};
},
methods: {
increment() {
this.counter++;
}
}
};
</script>
In this example, the increment
method is called when the button is clicked, which increases the value of the counter
data property. The template then updates to reflect the new value of counter
.
Computed Properties
Computed properties are like special reactive data properties. They are calculated based on other data properties. Crucially, computed properties are cached: if their dependencies don't change, the computed value is reused, which can improve performance.
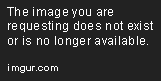
Example:
<template>
<div>
<h1>
Full Name: {{ fullName }}
</h1>
</div>
</template>
<script>
export default {
data() {
return {
firstName: 'John',
lastName: 'Doe'
};
},
computed: {
fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
};
</script>
Here, fullName
is a computed property that combines firstName
and lastName
. Whenever firstName
or lastName
changes, fullName
is automatically recalculated.
Key Differences Between Methods and Computed Properties
Here's a table summarizing the key differences:
Feature |
Methods |
Computed Properties |
---|---|---|
Purpose |
Actions, operations, side effects |
Calculated data, derived values |
Cache |
No cache |
Cached results (re-computed only if dependencies change) |
Reactivity |
Not directly reactive (only if they modify data) |
Reactive: Automatically re-calculate when dependencies change |
Execution |
Executed on demand (e.g., on button click) |
Executed automatically when dependencies change |
Side Effects |
Can have side effects (e.g., API calls, DOM manipulations) |
Generally should not have side effects (for performance and predictability) |
Use Cases and Examples
Methods
-
Event Handling:
When you need to perform actions in response to user events like button clicks, form submissions, or mouse interactions. -
Data Manipulation:
Modifying existing data, adding new items to arrays, or performing data transformations. -
API Interactions:
Making requests to external APIs to fetch or send data. -
DOM Manipulation:
Directly interacting with the DOM, though this should be done sparingly to preserve Vue's reactivity system.
Example: Submitting a Form
<template>
<form @submit.prevent="handleSubmit">
<input type="text" v-model="name"/>
<button type="submit">
Submit
</button>
</form>
</template>
<script>
export default {
data() {
return {
name: ''
};
},
methods: {
handleSubmit() {
// Send the name to the server using an API call
console.log('Submitting form with name:', this.name);
}
}
};
</script>
Computed Properties
-
Derived Data:
Calculating values based on other data properties. -
Filtering or Sorting:
Creating new views of data based on conditions. -
Conditional Logic:
Displaying different content based on calculated conditions. -
Optimization:
Preventing redundant calculations, improving performance by caching results.
Example: Displaying a Price with Taxes
<template>
<div>
Price: ${{ price }}
Tax: ${{ tax }}
Total: ${{ totalPrice }}
</div>
</template>
<script>
export default {
data() {
return {
price: 100,
taxRate: 0.05
};
},
computed: {
tax() {
return this.price * this.taxRate;
},
totalPrice() {
return this.price + this.tax;
}
}
};
</script>
In this example, tax
and totalPrice
are computed properties. They are automatically updated whenever price
or taxRate
changes, ensuring that the displayed values are always accurate.
Performance Considerations and Best Practices
-
Use Computed Properties for Performance:
Computed properties are cached, which can significantly improve performance, especially when dealing with complex calculations. -
Avoid Side Effects in Computed Properties:
Computed properties should primarily focus on calculated values and not have side effects like API calls or DOM manipulation. -
Avoid Over-Use of Methods:
Methods can be useful, but try to minimize their use if the logic can be implemented with a computed property for better performance. -
Optimize Large Lists:
When working with large lists, consider usingv-for
withkey
attributes for efficient updates and using computed properties to optimize list filtering and sorting.
Conclusion: When to Use Methods vs Computed Properties
Here's a simple guideline:
-
Methods:
For actions, operations, side effects, and event handling. -
Computed Properties:
For derived data, calculated values, optimization, and reactivity.
By understanding the differences and best practices for Methods and Computed Properties, you can write cleaner, more efficient Vue.js applications that deliver a smooth and responsive user experience.