<!DOCTYPE html>
Declarative vs Imperative Programming
<br>
body {<br>
font-family: Arial, sans-serif;<br>
line-height: 1.6;<br>
margin: 0;<br>
padding: 0;<br>
}</p>
<div class="highlight"><pre class="highlight plaintext"><code>h1, h2, h3 {
margin-bottom: 1rem;
}
code {
background-color: #f2f2f2;
padding: 0.2rem;
font-family: monospace;
}
img {
max-width: 100%;
display: block;
margin: 1rem auto;
}
.container {
max-width: 800px;
margin: 2rem auto;
padding: 1rem;
}
</code></pre></div>
<p>
Declarative vs Imperative Programming: A Comprehensive Guide
Introduction
Programming paradigms are fundamental approaches to software development that shape how we structure and express our code. Two prominent paradigms, declarative and imperative, offer contrasting perspectives on problem-solving. Understanding their differences is crucial for choosing the right paradigm for your project.
What is Declarative Programming?
Declarative programming focuses on what you want to achieve, rather than how to achieve it. You define the desired outcome, and the underlying implementation details are left to the language or framework.
Think of it as telling someone to "bake a chocolate cake." You don't specify the exact steps, ingredients, or baking time. You just state the goal, and they figure out the rest.

Key Characteristics of Declarative Programming:
- Focus on data and logic.
- No explicit control flow statements (loops, conditions).
- Often uses higher-order functions and data transformations.
- Less code, generally easier to understand and maintain.
- More expressive and concise.
What is Imperative Programming?
Imperative programming emphasizes the how aspect of problem-solving. It involves providing step-by-step instructions for the computer to execute, essentially outlining the exact sequence of actions needed to reach the desired outcome.
This is like giving someone a detailed recipe with precise instructions on mixing ingredients, baking times, and temperatures. You define every step needed to create the cake.
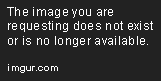
Key Characteristics of Imperative Programming:
- Focus on modifying state and controlling execution flow.
- Uses explicit control flow statements like loops, conditionals, and assignments.
- More code, potentially harder to understand and maintain.
- Greater control over execution and resource management.
Key Differences Between Declarative and Imperative Approaches
Feature |
Declarative |
Imperative |
---|---|---|
Focus |
What you want to achieve |
How to achieve it |
Control Flow |
Implicit |
Explicit |
State Management |
Often uses immutable data |
Explicitly modifies state |
Code Complexity |
Generally less complex |
Potentially more complex |
Readability |
Often easier to read and understand |
Can be more difficult to follow |
Parallelism |
Often more suitable for parallel processing |
May require careful synchronization for parallelism |
Examples |
SQL, HTML, CSS, Haskell, Prolog |
C, Java, Python (with mutable data structures) |
Examples of Declarative vs Imperative Code
Example 1: Sorting a List
Declarative (Python with sorted()
function):
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
Imperative (Python with a loop):
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5]
sorted_numbers = []
while numbers:
smallest = min(numbers)
sorted_numbers.append(smallest)
numbers.remove(smallest)
print(sorted_numbers)
Example 2: Data Filtering
Declarative (SQL):
SELECT * FROM customers WHERE age > 25;
Imperative (Java):
List
customers = getCustomers();
List
adultCustomers = new ArrayList<>();
for (Customer customer : customers) {
if (customer.getAge() > 25) {
adultCustomers.add(customer);
}
}
// Do something with adultCustomers
Benefits and Drawbacks of Each Paradigm
Declarative Programming
Benefits:
-
Simplicity and Readability
: Declarative code is often more concise and easier to understand, as it focuses on the outcome rather than the process. -
Maintainability
: Declarative code tends to be more maintainable due to its simplified structure and separation of concerns. -
Parallelism
: Declarative paradigms often lend themselves well to parallel processing due to the inherent focus on data transformations. -
Abstraction
: Declarative programming allows for a higher level of abstraction, hiding implementation details from the programmer.
Drawbacks:
-
Limited Control
: Less control over the underlying execution process, which might be undesirable in certain scenarios. -
Performance Concerns
: In some cases, declarative implementations might be less efficient than imperative ones, especially for complex operations. -
Limited Flexibility
: The abstraction provided by declarative programming can sometimes limit flexibility in specific scenarios.
Imperative Programming
Benefits:
-
Fine-grained Control
: Provides detailed control over the execution flow and resource management. -
Performance Optimization
: Allows for manual optimization of performance through careful control over algorithms and data structures. -
Widely Applicable
: Imperative programming is applicable to a wide range of problems and is well-suited for system-level programming.
Drawbacks:
-
Complexity
: Can lead to complex and difficult-to-understand code, especially for large-scale projects. -
Maintainability
: Imperative code can be harder to maintain, as changes in one part of the code might require cascading modifications elsewhere. -
Error Proneness
: The explicit control flow statements can lead to potential errors related to logic and sequencing.
Choosing the Right Paradigm for Your Project
The choice between declarative and imperative programming depends heavily on the specific project requirements and the nature of the problem you are trying to solve.
When to Choose Declarative Programming:
-
Data Transformation and Manipulation
: For tasks like filtering, sorting, and aggregating data, declarative approaches often excel. -
Readability and Maintainability
: When code clarity and ease of maintenance are paramount, declarative programming is a good choice. -
Concurrency and Parallelism
: For applications that require parallel processing, declarative paradigms can simplify concurrency management. -
Domain-Specific Languages (DSLs)
: Many DSLs are based on declarative principles, offering concise and expressive syntax for specific domains.
When to Choose Imperative Programming:
-
Low-Level Control
: When you need fine-grained control over resource management and execution flow, imperative programming provides the necessary flexibility. -
Performance Optimization
: For applications where performance is critical, imperative programming allows for manual optimization and fine-tuning. -
System-Level Programming
: For tasks like operating system development, device drivers, and embedded systems, imperative programming is often the preferred choice.
Conclusion
Declarative and imperative programming are two fundamental paradigms that offer distinct approaches to software development. Declarative programming emphasizes what you want to achieve, while imperative programming focuses on how to achieve it. Both paradigms have their own strengths and weaknesses, and the best choice depends on the specific project requirements.
For tasks involving data transformation, readability, and parallelism, declarative approaches can offer significant benefits. When you need fine-grained control, performance optimization, or system-level programming, imperative programming is often the more appropriate choice.
By understanding the differences between these paradigms and their respective advantages and disadvantages, you can make informed decisions about the best approach for your projects, leading to more efficient, maintainable, and effective software development.