<!DOCTYPE html>
Introduction to Python for Beginners
<br> body {<br> font-family: sans-serif;<br> margin: 0;<br> padding: 20px;<br> }<br> h1, h2, h3 {<br> color: #333;<br> }<br> code {<br> background-color: #f0f0f0;<br> padding: 2px 5px;<br> font-family: monospace;<br> }<br> pre {<br> background-color: #f0f0f0;<br> padding: 10px;<br> font-family: monospace;<br> overflow-x: auto;<br> }<br> img {<br> max-width: 100%;<br> height: auto;<br> display: block;<br> margin: 20px auto;<br> }<br>
Introduction to Python for Beginners
Welcome to the wonderful world of Python! This article will serve as your comprehensive guide to getting started with this versatile and powerful programming language. Whether you're a complete novice or have some coding experience, this introduction will provide you with a solid foundation to embark on your Python journey.
What is Python?
Python is a high-level, interpreted programming language known for its readability and ease of use. Its simple syntax and focus on code clarity make it an excellent choice for beginners. Python is widely used in various fields, including:
- Web development
- Data science and machine learning
- Scripting and automation
- Desktop applications
- Game development
- Artificial intelligence
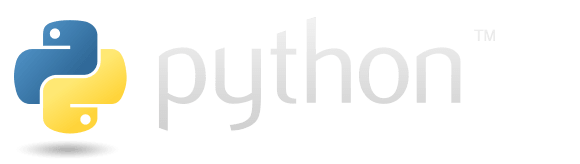
Setting up Your Python Environment
Before you can start writing Python code, you need to set up a Python development environment. Here's how:
- Download and Install Python
Visit the official Python website ( https://www.python.org/ ) and download the latest version of Python for your operating system (Windows, macOS, Linux). Follow the installer instructions to complete the installation process.
Open a terminal or command prompt and type
python --version
. If Python is installed correctly, you should see the version number displayed.
While you can write Python code using a simple text editor, using a code editor or an integrated development environment (IDE) is highly recommended. These tools offer features like syntax highlighting, code completion, debugging, and more. Some popular options include:
- Visual Studio Code (VS Code): https://code.visualstudio.com/
- PyCharm: https://www.jetbrains.com/pycharm/
- Sublime Text: https://www.sublimetext.com/
- Atom: https://atom.io/

Basic Python Syntax
Now that your environment is set up, let's dive into the fundamentals of Python syntax.
The
print()
function is your primary tool for displaying output in Python. Let's print a simple message:
print("Hello, world!")
This code will print the message "Hello, world!" to the console.
- Variables and Data Types
Variables are used to store data in your programs. They can be assigned values using the assignment operator (
=
). Here are some common data types:
- Integers (int): Whole numbers, e.g., 10, -5, 0
- Floating-point numbers (float): Numbers with decimal points, e.g., 3.14, -2.5
- Strings (str): Sequences of characters, e.g., "Hello", "Python"
- Booleans (bool): Truth values, e.g., True, False
# Assign values to variables
my_age = 25
pi = 3.14159
greeting = "Welcome to Python!"
is_python_cool = True
# Print the variable values
print(my_age)
print(pi)
print(greeting)
print(is_python_cool)
- Operators
Operators allow you to perform operations on variables and values. Here are some common operators:
- Arithmetic operators: +, -, , /, %, //, *
- Comparison operators: ==, !=, >, <, >=, <=
- Logical operators: and, or, not
- Assignment operators: =, +=, -=, *=, /=
# Arithmetic operations
result = 10 + 5
print(result) # Output: 15
# Comparison operations
is_equal = 10 == 10
print(is_equal) # Output: True
# Logical operations
is_true = True and False
print(is_true) # Output: False
- Control Flow
Control flow statements determine the order in which code is executed. Python provides various control flow constructs:
a) Conditional Statements (if-else)
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are not an adult yet.")
b) Looping Statements (for, while)
# For loop
for i in range(5):
print(i) # Output: 0 1 2 3 4
# While loop
count = 0
while count < 5:
print(count)
count += 1 # Increment count
Working with Data Structures
Python offers several built-in data structures that allow you to organize and manipulate data effectively.
- Lists
Lists are ordered collections of items. They are mutable, meaning their contents can be changed.
# Create a list
fruits = ["apple", "banana", "cherry"]
# Access elements by index
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
# Modify list elements
fruits[1] = "grape"
# Add elements
fruits.append("mango")
# Remove elements
fruits.remove("apple")
# Print the modified list
print(fruits) # Output: ['banana', 'grape', 'cherry', 'mango']
- Dictionaries
Dictionaries are key-value pairs. They are also mutable.
# Create a dictionary
student = {
"name": "John Doe",
"age": 20,
"major": "Computer Science"
}
# Access values by key
print(student["name"]) # Output: John Doe
print(student["age"]) # Output: 20
# Modify values
student["age"] = 21
# Add new key-value pairs
student["city"] = "New York"
# Print the modified dictionary
print(student)
- Functions
Functions are reusable blocks of code that perform specific tasks. They help you organize and modularize your programs.
def greet(name):
"""Greets the user by name."""
print("Hello,", name, "!")
# Call the function
greet("Alice") # Output: Hello, Alice !
Conclusion
Congratulations! You've taken your first steps into the world of Python. You've learned the basics of syntax, how to work with variables, data types, operators, control flow, and fundamental data structures like lists and dictionaries. You've also explored the power of functions to create reusable code blocks.
This is just the beginning of your Python journey. There's a vast world of possibilities to explore: web development frameworks like Django and Flask, data science libraries like NumPy, Pandas, and Scikit-learn, and much more. As you continue your learning, remember these key takeaways:
-
Practice regularly:
The more you write Python code, the more comfortable you'll become. -
Experiment and explore:
Don't be afraid to try new things and experiment with different concepts. -
Seek help when needed:
Online communities, forums, and documentation are valuable resources for finding answers to your questions. -
Have fun!:
Programming should be enjoyable, so embrace the challenge and enjoy the process of learning.
Keep coding, and happy Pythoning!