<!DOCTYPE html>
JavaScript Truthy and Falsy Values
<br> body {<br> font-family: sans-serif;<br> margin: 20px;<br> }</p> <div class="highlight"><pre class="highlight plaintext"><code> h1, h2, h3 { color: #333; } pre { background-color: #eee; padding: 10px; border-radius: 5px; font-size: 16px; } code { font-family: monospace; } img { max-width: 100%; height: auto; display: block; margin: 20px auto; } </code></pre></div> <p>
JavaScript Truthy and Falsy Values
In JavaScript, every value has an associated truthiness. This means that each value can be evaluated as either
true
or
false
in a boolean context. This concept is crucial for conditional statements (
if
,
else
), loops (
while
,
for
), and logical operators (
&&
,
||
,
!
).
Truthy and Falsy Values
Here's a breakdown of the values that are considered falsy (evaluated as
false
) in JavaScript:
-
false
-
(the number zero)
0
-
(an empty string)
""
-
null
-
undefined
-
(Not a Number)
NaN
All other values are considered truthy (evaluated as
true
). This includes:
-
true
- All non-zero numbers, including negative numbers
- Non-empty strings
- Arrays (even empty arrays)
- Objects (even empty objects)
- Functions
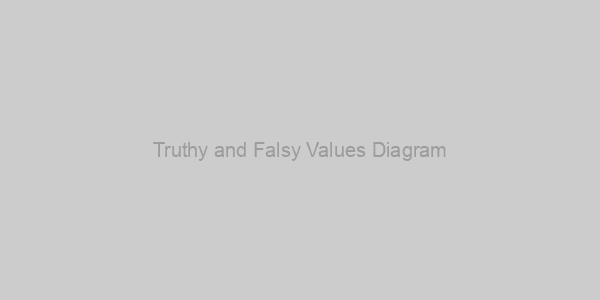
Understanding Truthy and Falsy in Practice
Let's look at some examples to see how truthy and falsy values work in action.
Conditional Statements
// Example 1: Truthy value
if (1) {
console.log("This will always execute because 1 is truthy.");
}
// Example 2: Falsy value
if (0) {
console.log("This will never execute because 0 is falsy.");
}
// Example 3: Truthy value
let myString = "Hello";
if (myString) {
console.log("This will execute because 'Hello' is truthy.");
}
// Example 4: Falsy value
let emptyArray = [];
if (emptyArray.length === 0) {
console.log("The empty array is falsy.");
}
Logical Operators
// Example 1: Logical AND (&&)
let age = 18;
let isAdult = age >= 18 && true; // Both conditions are truthy, result is true
console.log(isAdult); // Output: true
// Example 2: Logical OR (||)
let hasPermission = false;
let canAccess = hasPermission || age >= 18; // One condition is truthy, result is true
console.log(canAccess); // Output: true
// Example 3: Logical NOT (!)
let isActive = true;
let isInactive = !isActive; // Flips the boolean value to false
console.log(isInactive); // Output: false
Loops
// Example 1: While loop with truthy condition
let counter = 1;
while (counter <= 5) {
console.log(counter);
counter++;
}
// Example 2: For loop with truthy condition
for (let i = 0; i < 3; i++) {
console.log("Iteration:", i);
}
Why Truthy and Falsy Are Important
-
Code Readability and Conciseness: Truthy and falsy values allow you to write more compact and expressive code. Instead of explicitly checking for equality with
true
or
false
, you can simply use the value itself in a boolean context.- Flexibility in Conditional Logic: Truthy and falsy values provide flexibility in handling various data types and states within conditional statements. You can check for the presence or absence of data, the existence of objects, or the truthiness of complex expressions without writing verbose comparisons.
-
Understanding Function Arguments: Some functions in JavaScript (e.g.,
Array.prototype.some()
,Array.prototype.every()
,Boolean()
) rely on the truthiness of values passed as arguments.Best Practices
-
Explicit Comparisons When Necessary: While truthy and falsy values can be convenient, it's important to use explicit comparisons (
or
!==
) when precise equality is crucial to avoid unexpected behavior.- Avoid Confusing Behavior: Be mindful of situations where the intended logic might be unclear. Use explicit comparisons to clarify the intent, especially if the code needs to be easily understood by others.
- Know Your Data Types: Understand the truthiness of different data types to ensure your code behaves as expected.
-
Be Aware of Potential Errors: Falsy values can sometimes lead to unintended consequences. Be mindful of potential errors when using truthy and falsy values, and use explicit checks when necessary.
Conclusion
Truthy and falsy values are fundamental concepts in JavaScript, providing a powerful mechanism for simplifying conditional logic and enhancing code readability. By understanding the various values that evaluate astrue
orfalse
in a boolean context, you can write more effective and concise JavaScript code. Remember to use explicit comparisons when necessary to ensure clarity and avoid potential errors, and always prioritize code readability for better maintainability.