<!DOCTYPE html>
In-Memory Databases with SQLite
<br>
body {<br>
font-family: Arial, sans-serif;<br>
margin: 0;<br>
padding: 20px;<br>
}<br>
h1, h2, h3 {<br>
color: #333;<br>
}<br>
pre {<br>
background-color: #f5f5f5;<br>
padding: 10px;<br>
border-radius: 5px;<br>
font-family: monospace;<br>
}<br>
img {<br>
max-width: 100%;<br>
display: block;<br>
margin: 0 auto;<br>
}<br>
In-Memory Databases with SQLite
Introduction
SQLite is a widely used, file-based, embedded database system. Its lightweight nature and ease of use have made it a popular choice for various applications, including mobile apps, desktop software, and web applications. While SQLite typically stores data on disk, it also offers a powerful feature: in-memory databases. This allows you to create a database that exists solely in your application's memory, providing significant performance gains for certain use cases.
This article delves into the world of in-memory databases with SQLite, exploring its advantages, limitations, and practical implementation.
Understanding In-Memory Databases
A traditional database stores data on a persistent storage medium like a hard drive. Every time data needs to be accessed, the database engine has to read it from disk, which can be time-consuming, especially for large datasets. In contrast, an in-memory database stores its entire contents within the application's RAM. This means data access becomes significantly faster as data retrieval and manipulation happen directly in memory, eliminating the overhead of disk operations.
Here's a visual representation of the difference:
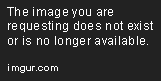
Benefits of In-Memory SQLite
In-memory SQLite databases offer several compelling advantages:
-
Exceptional Performance:
In-memory databases significantly reduce data access times, leading to faster application performance, especially for applications with frequent database interactions. -
Simple Implementation:
SQLite's ease of use extends to in-memory databases. You can create and use them with minimal code changes. -
Flexibility:
In-memory databases provide a dynamic environment for data manipulation. You can create, update, and delete data without the constraints of persistent storage. -
Ideal for Temporary Data:
If your application handles data that's transient or only needed during a session, in-memory SQLite offers a highly efficient solution.
Limitations of In-Memory SQLite
While in-memory databases provide impressive performance, they also have limitations:
-
Data Persistence:
In-memory databases vanish when the application closes. Any data stored in them is lost. If persistence is needed, you'll need to employ techniques like saving data to disk before closing. -
Memory Consumption:
Large datasets can consume considerable memory, potentially affecting application performance or leading to memory exhaustion. -
Limited Functionality:
Some features, like foreign keys and triggers, may behave differently or have limitations when used with in-memory databases. It's essential to consult SQLite documentation for specific behavior.
Implementation Guide
Creating an In-Memory Database
Creating an in-memory SQLite database is straightforward. You simply specify a database name that starts with ":memory:".
import sqlite3# Create an in-memory database conn = sqlite3.connect(':memory:') # Create a table conn.execute('''CREATE TABLE customers ( id INTEGER PRIMARY KEY, name TEXT, email TEXT )''') # Insert some data conn.execute("INSERT INTO customers (name, email) VALUES ('Alice', 'alice@example.com')") conn.execute("INSERT INTO customers (name, email) VALUES ('Bob', 'bob@example.com')") # Retrieve data cursor = conn.execute("SELECT * FROM customers") for row in cursor: print(row) # Close the connection conn.close()
Using the In-Memory Database
Once you've created an in-memory database, you can interact with it using the same SQLite API you'd use for a disk-based database. You can perform operations like:
- Creating, deleting, and modifying tables
- Inserting, updating, and deleting data
- Querying data using SQL statements
Handling Persistence
Since in-memory databases lack persistence, you need to implement mechanisms to save data if you want to retain it across application sessions. Here are some approaches:
-
Periodically Save to Disk:
You can periodically save the database contents to a file using thesqlite3.connect
function with a file path instead of ":memory:". -
Save on Application Exit:
Implement a mechanism to save the database to disk when the application closes. -
Use a Hybrid Approach:
Combine an in-memory database for fast operations with a disk-based database for persistence. You can periodically synchronize data between the two.
Example: In-Memory Shopping Cart
Let's demonstrate the use of in-memory SQLite for a simple shopping cart application:
import sqlite3# Create an in-memory database conn = sqlite3.connect(':memory:') # Create a table for cart items conn.execute('''CREATE TABLE cart ( id INTEGER PRIMARY KEY, product_name TEXT, price REAL, quantity INTEGER )''') # Add items to the cart def add_item(product_name, price, quantity): conn.execute("INSERT INTO cart (product_name, price, quantity) VALUES (?, ?, ?)", (product_name, price, quantity)) # Remove an item from the cart def remove_item(product_name): conn.execute("DELETE FROM cart WHERE product_name = ?", (product_name,)) # Get the cart total def get_cart_total(): cursor = conn.execute("SELECT SUM(price * quantity) FROM cart") total = cursor.fetchone()[0] return total if total is not None else 0 # ... (More cart operations like updating quantities) # Example usage add_item("Apple", 1.0, 2) add_item("Banana", 0.5, 3) print("Cart Total:", get_cart_total())
Conclusion
In-memory SQLite databases offer a powerful solution for applications demanding high performance, especially for transient data or when disk I/O is a bottleneck. Their ease of implementation and integration with the SQLite API make them a convenient choice for various scenarios.
While in-memory databases provide incredible speed, they come with limitations. Understanding their data persistence behavior and memory consumption is crucial to avoid potential issues. By carefully considering these factors, you can harness the benefits of in-memory SQLite to optimize your application's performance and user experience.