<!DOCTYPE html>
Flutter PopupMenuButton: A Comprehensive Guide
<br>
body {<br>
font-family: Arial, sans-serif;<br>
line-height: 1.6;<br>
margin: 0;<br>
padding: 0;<br>
background-color: #f4f4f4;<br>
}</p>
<div class="highlight"><pre class="highlight plaintext"><code> .container {
max-width: 800px;
margin: 20px auto;
padding: 20px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1, h2, h3, h4 {
color: #333;
}
code {
background-color: #f0f0f0;
padding: 2px 5px;
font-family: monospace;
}
pre {
background-color: #f0f0f0;
padding: 10px;
border-radius: 5px;
overflow-x: auto;
}
img {
max-width: 100%;
height: auto;
display: block;
margin: 20px auto;
}
.image-container {
text-align: center;
}
</code></pre></div>
<p>
Flutter PopupMenuButton: A Comprehensive Guide
In the realm of Flutter app development, providing users with convenient and intuitive interactions is paramount. One way to achieve this is through the use of
PopupMenus
, which allow users to access a list of options in a compact and user-friendly manner. This guide delves into the intricacies of Flutter's
PopupMenuButton
widget, equipping you with the knowledge and skills to effectively implement this powerful feature in your applications.
Introduction to Flutter PopupMenuButton
The
PopupMenuButton
widget in Flutter enables you to present a dropdown menu with a set of options to users. This menu typically appears when a user taps on a button or other trigger element. It offers a concise and organized way to provide users with a selection of actions or settings, enhancing the user experience by minimizing screen clutter.
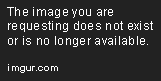
Essential Concepts
Before diving into the implementation details, let's grasp some core concepts associated with the
PopupMenuButton
:
1. Properties
-
child:
The widget displayed as the trigger for the PopupMenu. It could be a button, icon, or any other widget. -
onSelected:
A callback function invoked when an item from the PopupMenu is selected. This function receives the value associated with the selected item. -
itemBuilder:
A builder function that dynamically creates the items to be displayed within the PopupMenu. It takes a
as input and returns a list of
BuildContext
widgets.
PopupMenuEntry
-
offset:
An
object specifying the position of the PopupMenu relative to the trigger widget.
Offset
-
elevation:
A value that controls the shadow intensity of the PopupMenu. -
padding:
Padding applied to the inner content of the PopupMenu.
2. PopupMenuEntry
Each item in a
PopupMenu
is represented by a
PopupMenuEntry
widget. There are various subtypes of
PopupMenuEntry
, each tailored to specific needs:
-
PopupMenuItem:
Represents a simple menu item with text and an optional value. It can be customized with properties like
,
value
, and
enabled
.
textStyle
-
CheckedPopupMenuItem:
Similar to
but allows you to visually indicate a checked/selected state. It has an additional property
PopupMenuItem
to track the selection.
checked
-
Divider:
A simple horizontal divider to separate items within the PopupMenu.
Step-by-Step Implementation
Now, let's illustrate how to use
PopupMenuButton
in a Flutter application. We'll create a simple example to demonstrate its functionality:
1. Create a Widget
import 'package:flutter/material.dart';class MyPopupMenuWidget extends StatelessWidget { const MyPopupMenuWidget({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Center( child: PopupMenuButton<string>( onSelected: (value) { // Handle selected value here print('Selected: $value'); }, itemBuilder: (context) { return [ PopupMenuItem( value: 'Item 1', child: Text('Item 1'), ), PopupMenuItem( value: 'Item 2', child: Text('Item 2'), ), PopupMenuItem( value: 'Item 3', child: Text('Item 3'), ), ]; }, child: Icon(Icons.more_vert), ), ); } } </string></pre>
2. Run the Application
In your
main.dart
file, use the
MyPopupMenuWidget
within a
Scaffold
or any other relevant layout structure:
import 'package:flutter/material.dart';
import 'package:flutter_app/my_popup_menu_widget.dart'; // Import your widgetvoid main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'PopupMenuButton Demo', home: Scaffold( appBar: AppBar(title: Text('PopupMenuButton Demo')), body: MyPopupMenuWidget(), // Use your widget here ), ); } } </pre>
By running this code, you will see an icon with three vertical dots. Tapping on this icon will reveal the PopupMenu with the defined options. When an item is selected, the
onSelected
callback will be triggered, printing the selected value to the console.
Advanced Features
The
PopupMenuButton
offers several advanced features to customize its behavior and appearance:
1. Customizing the Trigger
Instead of using the default icon, you can customize the trigger widget. For example, you could use a
Text
widget to display a label:
PopupMenuButton(
// ... other properties
child: Text('Select an Option'),
)
2. Handling Checked Items
To indicate a checked state for an item, use
CheckedPopupMenuItem
. The
checked
property determines whether the item is checked. You can manage the checked state using a variable and update it within the
onSelected
callback:
bool _item1Checked = false;// Inside the itemBuilder function: return [ CheckedPopupMenuItem( value: 'Item 1', checked: _item1Checked, child: Text('Item 1'), ), // ... other items ]; // Inside the onSelected callback: onSelected: (value) { if (value == 'Item 1') { _item1Checked = !_item1Checked; } // ... other logic }, </pre>
3. Using a Custom Offset
You can control the position of the PopupMenu by specifying an
offset
. This is particularly useful when you want to adjust the menu's placement relative to the trigger widget:
PopupMenuButton(
// ... other properties
offset: Offset(10.0, 10.0), // Move 10 pixels to the right and down
)
4. Adding a Custom Padding
The
padding
property allows you to add space around the menu items. You can set a uniform padding or use
EdgeInsets
to customize padding for specific sides:
PopupMenuButton(
// ... other properties
padding: EdgeInsets.all(16.0), // Add 16 pixels padding around all sides
)
Best Practices
Here are some best practices to ensure effective use of
PopupMenuButton
in your Flutter apps:
-
Keep Menu Options Concise:
Limit the number of options in the PopupMenu to avoid overwhelming users. -
Use Clear and Descriptive Labels:
Make sure each menu item has a clear and concise label that accurately describes its function. -
Consider Accessibility:
Use appropriate colors and contrasts to make the PopupMenu accessible to users with visual impairments. Ensure that the menu is properly labeled for screen readers. -
Provide Feedback:
Clearly indicate to the user which option has been selected, perhaps through visual cues or a confirmation message.
Conclusion
The
PopupMenuButton
widget in Flutter provides a powerful and versatile way to present a dropdown menu with options to users. By understanding its properties,
PopupMenuEntry
subtypes, and advanced features, you can effectively incorporate this widget into your Flutter apps to enhance user interaction and provide a seamless user experience. Remember to follow best practices to ensure accessibility, clarity, and an intuitive menu design.