<!DOCTYPE html>
React Query: Making Your Server-State Problems Disappear (Like Magic)
<br> body {<br> font-family: sans-serif;<br> }<br> h1, h2, h3 {<br> margin-top: 30px;<br> }<br> pre {<br> background-color: #f0f0f0;<br> padding: 10px;<br> border-radius: 5px;<br> }<br> img {<br> max-width: 100%;<br> }<br>
React Query: Making Your Server-State Problems Disappear (Like Magic)
Building modern web applications often involves fetching data from external APIs or databases. This server-side data is essential for rendering dynamic content, providing interactive features, and keeping your application up-to-date. However, managing this data flow in React can be a complex and error-prone process.
Enter React Query, a powerful library that takes the pain out of server-state management in React applications. By providing a robust framework for fetching, caching, and updating data, React Query simplifies your code, improves performance, and enhances the user experience. This article will guide you through the magical world of React Query, empowering you to handle server-state with ease.
Why React Query?
React Query tackles a range of challenges that developers encounter when dealing with server-state:
-
Data Fetching:
React Query streamlines the process of retrieving data from external sources. It provides a clear and consistent API for making requests, handling responses, and managing errors. -
Caching:
React Query automatically caches data responses, reducing the need for unnecessary network requests. This improves performance, especially when dealing with frequently accessed data. -
Data Synchronization:
React Query ensures data consistency across your application by automatically updating components when data changes. It also handles potential race conditions and stale data issues. -
State Management:
React Query simplifies state management by providing a dedicated store for server-side data. This eliminates the need to manually track and manage data within your components. -
Error Handling:
React Query offers comprehensive error handling mechanisms, allowing you to gracefully handle network failures, API errors, and other potential issues. -
Background Updates:
React Query can automatically refresh data in the background, ensuring that your application always displays the latest information. -
Optimistic Updates:
React Query allows you to implement optimistic updates, providing a more seamless user experience by immediately updating the UI even before the server response is received.
By addressing these common issues, React Query empowers you to build more efficient, responsive, and user-friendly applications.
A Deep Dive into React Query
Let's delve into the key concepts and features of React Query:
- Queries
Queries are the core of React Query. They define how data is fetched from an API or database. Each query is associated with a unique key, allowing React Query to identify and manage it.
import { useQuery } from 'react-query';const fetchPosts = async () => {
const response = await fetch('https://api.example.com/posts');
return response.json();
};function PostList() {
const { isLoading, error, data } = useQuery('posts', fetchPosts);if (isLoading) return
Loading...
;
if (error) returnError: {error.message}
;return (
-
{data.map((post) => (
- {post.title} ))}
);
}
In this example,
useQuery
creates a query named "posts" using the
fetchPosts
function. React Query automatically fetches the data, manages its state, and updates the component accordingly.
- Caching
React Query automatically caches the results of each query. Subsequent requests for the same data will be retrieved from the cache, improving performance and reducing the number of network requests.
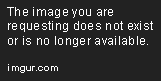
You can control the caching behavior using options such as
staleTime
(how long data remains valid in the cache) and
cacheTime
(how long data is kept in the cache even after it's stale).
React Query also supports mutations, which allow you to modify data on the server. Mutations are similar to queries, but they initiate an action (e.g., creating, updating, or deleting data).
import { useMutation } from 'react-query';
const createPost = async (post) => {
const response = await fetch('https://api.example.com/posts', {
method: 'POST',
body: JSON.stringify(post),
});
return response.json();
};
function PostForm() {
const [createPostMutation, { isLoading, error, data }] = useMutation(
createPost,
);
const handleSubmit = (event) => {
event.preventDefault();
const post = { title: 'New Post' };
createPostMutation(post);
};
if (isLoading) return
Saving...
;if (error) return
Error: {error.message}
;return (
Create Post
);
}
In this example,
useMutation
defines a mutation called "createPost" that uses the
createPost
function to send data to the server. When the form is submitted, the mutation is executed, updating the data and triggering the component to re-render.
- Query Invalidation
React Query provides mechanisms for invalidating queries when data changes. This ensures that components always display the latest information.
-
Manual Invalidation:
You can manually invalidate a query using the
queryClient.invalidateQueries()
method. -
Automatic Invalidation:
React Query can automatically invalidate queries when mutations are successful. This is achieved by specifying the query keys that should be invalidated within the
onMutate
andonSuccess
callbacks of the mutation.
React Query allows you to implement optimistic updates, improving the user experience by immediately updating the UI even before the server response is received.
import { useMutation } from 'react-query';
const updatePost = async (post) => {
const response = await fetch(https://api.example.com/posts/${post.id}
, {
method: 'PUT',
body: JSON.stringify(post),
});
return response.json();
};
function PostEditor({ post }) {
const [updatePostMutation] = useMutation(updatePost, {
onMutate: (newPost) => {
// Update the UI immediately
return newPost;
},
});
const handleSave = () => {
updatePostMutation(post);
};
return (
{/* Input fields for editing post data */}
Save Changes
);
}
In this example, the
onMutate
callback updates the UI with the new post data immediately, while the actual server update is being processed. This provides instant feedback to the user, making the application feel more responsive.
- Other Features
React Query offers several additional features that enhance its usability and functionality:
- Parallel Queries: React Query allows you to fetch multiple queries simultaneously, improving performance.
- Query Dependencies: You can define dependencies between queries, ensuring that a query is re-executed when its dependent query changes.
- Focus Tracking: React Query automatically suspends queries when the user navigates away from the relevant component, reducing unnecessary network requests.
- Customizability: React Query is highly customizable, allowing you to tailor its behavior to fit your specific needs.
Step-by-Step Guide: Building a Simple App with React Query
Let's put React Query into action by building a simple application that fetches and displays a list of users:
npm install react-query
import { useQuery } from 'react-query';const fetchUsers = async () => {
const response = await fetch('https://api.example.com/users');
return response.json();
};
3. Implement the user list component:
function UserList() {
const { isLoading, error, data } = useQuery('users', fetchUsers);if (isLoading) return
Loading users...
;
if (error) returnError: {error.message}
;return (
-
{data.map((user) => (
- {user.name} ))}
);
}
4. Render the component:
function App() {
return (
User List
);
}
This example demonstrates how to use React Query to fetch and display data from an API. The
useQuery
hook handles data fetching, caching, and state management, simplifying your code and improving performance.
Best Practices
To get the most out of React Query, follow these best practices:
-
Use meaningful query keys:
Choose descriptive keys that reflect the data being fetched. This makes your code more readable and easier to debug. -
Cache data strategically:
Use the
and
staleTime
options to control how long data is kept in the cache. Consider the frequency of data updates and the importance of displaying the latest information.
cacheTime
-
Optimize performance:
Use the
option to prevent unnecessary queries. Consider using query dependencies to re-execute queries only when necessary.
enabled
-
Handle errors gracefully:
Implement robust error handling mechanisms to provide a seamless user experience in case of network failures or API errors. -
Use optimistic updates when appropriate:
Consider implementing optimistic updates for actions that modify data. This improves user experience by providing instant feedback.
Conclusion
React Query is a game-changer for server-state management in React applications. It simplifies complex tasks, enhances performance, and provides a robust framework for handling data flow. By leveraging its power, you can build more efficient, responsive, and user-friendly applications with ease. This article has provided a comprehensive overview of React Query, covering its key concepts, features, and best practices. Get started with React Query today and witness the magic it brings to your server-state management!