<!DOCTYPE html>
Talking to a Gmail POP3 Server with Python
<br> body {<br> font-family: Arial, sans-serif;<br> line-height: 1.6;<br> margin: 0;<br> padding: 0;<br> }</p> <div class="highlight"><pre class="highlight plaintext"><code> h1, h2, h3 { color: #333; } code { background-color: #f5f5f5; padding: 2px 5px; font-family: monospace; } pre { background-color: #f5f5f5; padding: 10px; border: 1px solid #ddd; overflow-x: auto; } img { max-width: 100%; height: auto; display: block; margin: 10px auto; } </code></pre></div> <p>
Talking to a Gmail POP3 Server with Python
In the world of email, POP3 (Post Office Protocol version 3) is a long-standing standard for retrieving emails from a server. While IMAP (Internet Message Access Protocol) has largely taken over for its ability to synchronize email data across devices, POP3 still holds its own for tasks like downloading emails for offline access or integrating email functionality into Python applications. This article will guide you through the process of connecting to a Gmail POP3 server using Python, retrieving emails, and interacting with their contents.
Why Use POP3?
Before diving into the code, let's understand the scenarios where using POP3 with Gmail might be beneficial:
-
Offline Email Access:
POP3 lets you download emails to your device, allowing you to access them without an internet connection. This can be helpful for mobile devices or areas with unreliable internet access. -
Integrating Email with Python Applications:
POP3 simplifies fetching email data for processing within Python scripts. This is useful for tasks like email parsing, automation, or building email-based systems. -
Simple Email Retrieval:
For straightforward email retrieval tasks, POP3 offers a less complex approach compared to IMAP's synchronization capabilities.
Setting up Gmail for POP3
Before you can access your Gmail account through POP3, you need to configure your Gmail settings:
-
Enable POP3 access:
Log in to your Gmail account and navigate to
Settings > Forwarding and POP/IMAP
. -
Choose the POP setting:
Select "Enable POP for all mail" or "Enable POP for mail that arrives from now on". This will determine whether POP3 retrieves all emails or only new ones. -
Save Changes:
Click "Save Changes" at the bottom of the page.
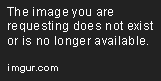
Python Libraries
We will be using the
poplib
library, which is part of the Python standard library, for interacting with the POP3 server.
Code Walkthrough
Let's break down the Python code for connecting to a Gmail POP3 server, retrieving emails, and extracting data.
import poplib
import email
def connect_to_pop3(email_address, password):
"""Connects to the Gmail POP3 server and returns the connection object."""
# Connect to the POP3 server
pop_server = poplib.POP3_SSL('pop.gmail.com')
# Log in with your email address and password
pop_server.user(email_address)
pop_server.pass_(password)
return pop_server
def retrieve_emails(pop_server):
"""Retrieves all emails from the server and returns them as a list."""
# Get the number of emails in the mailbox
num_emails = len(pop_server.list()[1])
# Create a list to store the email messages
emails = []
# Iterate through each email
for i in range(1, num_emails + 1):
# Retrieve the email message
_, lines, _ = pop_server.retr(i)
email_body = "\n".join(lines)
# Convert the email body into an email object
email_msg = email.message_from_string(email_body)
# Add the email message to the list
emails.append(email_msg)
return emails
def extract_email_data(email_msg):
"""Extracts relevant data from an email message."""
# Get the email subject
subject = email_msg['Subject']
# Get the sender's email address
sender = email_msg['From']
# Get the message body
body = email_msg.get_payload()
return subject, sender, body
def main():
"""Main function to connect, retrieve emails, and extract data."""
# Replace with your email address and password
email_address = 'your_email@gmail.com'
password = 'your_password'
# Connect to the POP3 server
pop_server = connect_to_pop3(email_address, password)
# Retrieve emails from the server
emails = retrieve_emails(pop_server)
# Process each email
for email_msg in emails:
subject, sender, body = extract_email_data(email_msg)
# Print the email details
print(f"Subject: {subject}")
print(f"Sender: {sender}")
print(f"Body: {body}")
# Optionally, perform further processing or store the email data
# Disconnect from the server
pop_server.quit()
if name == "main":
main()
Explanation
-
Import necessary libraries:
poplib
for handling POP3 interactions.
email
for parsing email messages.- Connect to the POP3 server:
- The
connect_to_pop3
function establishes a secure connection to the Gmail POP3 server usingpoplib.POP3_SSL('pop.gmail.com')
. - It then authenticates with your email address and password.
3. Retrieve emails:
retrieve_emails
gets the number of emails in your inbox using
pop_server.list()
.- It iterates through each email, retrieves its raw data, converts it to an
email.message
object, and adds it to a list.
4. Extract email data:
extract_email_data
takes an
email.message
object and extracts the subject, sender, and message body.- Main function:
- The
main
function calls the previous functions to connect to the server, retrieve emails, and process each email. - It prints the extracted email details for demonstration.
- Remember to replace the placeholder email address and password with your credentials.
Important Considerations
- Security: Always use secure connections (POP3_SSL) and handle email credentials responsibly. Consider using environment variables or secure storage methods to protect your password.
- Email Deletion:** By default, POP3 typically marks emails as deleted on the server after retrieving them. This behavior can be modified in your Gmail settings or using POP3 commands.
- Email Content: Emails often contain attachments, HTML formatting, and other complex components. Handling these aspects requires additional parsing and processing.
- Gmail Limits: Gmail may have limits on how many emails can be downloaded using POP3 within a certain timeframe.
Conclusion
Using POP3 with Gmail and Python can be a powerful tool for retrieving emails for various applications. Understanding the underlying concepts, setting up your Gmail account for POP3 access, and leveraging the
poplib
library enables you to fetch emails, process their contents, and build automated email workflows. Remember to prioritize security and understand the implications of email deletion when working with POP3.