<!DOCTYPE html>
Understanding the Difference Between ClassNotFoundException and NoClassDefFoundError
<br> body {<br> font-family: Arial, sans-serif;<br> margin: 0;<br> padding: 0;<br> }</p> <div class="highlight"><pre class="highlight plaintext"><code>h1, h2, h3 { text-align: center; margin-top: 20px; } p { text-align: justify; line-height: 1.6; margin: 10px; } code { background-color: #f0f0f0; padding: 5px; border-radius: 5px; } pre { background-color: #f0f0f0; padding: 10px; border-radius: 5px; overflow: auto; } img { display: block; margin: 20px auto; } </code></pre></div> <p>
Understanding the Difference Between ClassNotFoundException and NoClassDefFoundError
In the world of Java programming, exceptions are an integral part of the development process. These exceptions, while initially appearing as errors, provide valuable information about the unexpected behavior of your code. Among these exceptions, two that often cause confusion are the
ClassNotFoundException
and
NoClassDefFoundError
. While they both indicate that the Java Virtual Machine (JVM) cannot locate a class, they differ significantly in their origins and the actions required to resolve them.
Introduction
Both
ClassNotFoundException
and
NoClassDefFoundError
are runtime exceptions, thrown when the JVM encounters an issue related to the loading of a class file. Understanding the distinction between these exceptions is essential for debugging and resolving these issues effectively.
Deep Dive
ClassNotFoundException: The Class is Not Found at Runtime
The
ClassNotFoundException
occurs when the JVM tries to load a class during runtime, but the class file is not found in the classpath. This happens when:
- The class file is missing from the classpath.
- The class name is misspelled or incorrect.
-
The class is dynamically loaded using
, but the class file is not available.
Class.forName()
Here's a simple example:
public class ClassNotFoundExample {
public static void main(String[] args) {
try {
Class.forName("com.example.MyMissingClass");
} catch (ClassNotFoundException e) {
System.out.println("ClassNotFoundException: " + e.getMessage());
}
}
}
In this example,
com.example.MyMissingClass
is not found in the classpath, resulting in a
ClassNotFoundException
.
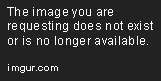
NoClassDefFoundError: The Class was Found at Compile Time, But Not at Runtime
The
NoClassDefFoundError
arises when a class was successfully compiled and found at compile time, but the JVM cannot locate the class file at runtime. This could be due to:
- The class file was removed or moved from the classpath after compilation.
- There's a problem with the class loader hierarchy.
- A dependency is missing from the runtime environment.
Consider the following scenario:
public class NoClassDefFoundExample {
public static void main(String[] args) {
// ExampleClass is compiled against a JAR file
ExampleClass exampleObject = new ExampleClass();
}
}
If the JAR file containing
ExampleClass
is removed from the classpath after compilation, the JVM will throw a
NoClassDefFoundError
at runtime.

Key Differences
| Feature | ClassNotFoundException | NoClassDefFoundError |
|---|---|---|
| Origin | Class not found at runtime | Class found at compile time but not at runtime |
| Cause | Missing or invalid class file | Missing class file at runtime, usually due to classpath issues or dependency problems |
| Error Type | Runtime exception | Error |
| Solution | Verify classpath, check for typos, ensure class file exists | Fix classpath, check dependencies, verify JAR files are present |
Step-by-Step Guides and Examples
Resolving ClassNotFoundException
Here's a step-by-step guide to resolve
ClassNotFoundException
:
-
Verify Classpath: Make sure the classpath includes the location where the missing class file is present. Check the classpath settings in your IDE or build environment.
- Check for Typos: Carefully review the class name in your code for any spelling errors or incorrect capitalization.
- Ensure Class File Availability: Ensure that the class file exists in the specified location and is not corrupted.
-
Dynamic Loading with Class.forName(): If using
Class.forName()
, ensure the class file is available at runtime. You can use a custom class loader or place the class file in a location accessible to the JVM.Resolving NoClassDefFoundError
To resolve
NoClassDefFoundError
, follow these steps:
-
Check Classpath: Verify that the classpath contains the necessary JAR files or directories where the missing class is located.
- Review Dependencies: Ensure all dependencies required by the application are included in the project's build configuration and are present in the runtime environment.
- Check for Corrupted JAR Files: Inspect the JAR files for any corruption or inconsistencies.
-
Dependency Management: Use a dependency management tool like Maven or Gradle to handle the dependencies automatically and ensure they are available at runtime.
Conclusion
Understanding the difference between
ClassNotFoundException
andNoClassDefFoundError
is crucial for Java developers. They indicate separate problems related to class loading and require distinct solutions. By carefully examining the classpath, dependencies, and runtime environment, developers can effectively diagnose and resolve these issues, ensuring smooth application execution.