<!DOCTYPE html>
Constructors in Java: Building Objects from the Ground Up
<br> body {<br> font-family: sans-serif;<br> line-height: 1.6;<br> margin: 0;<br> padding: 20px;<br> }</p> <div class="highlight"><pre class="highlight plaintext"><code> h1, h2, h3 { margin-top: 2em; } pre { background-color: #f5f5f5; padding: 10px; border-radius: 5px; overflow-x: auto; } code { font-family: monospace; } img { max-width: 100%; height: auto; display: block; margin: 20px auto; } </code></pre></div> <p>
Constructors in Java: Building Objects from the Ground Up
In the world of object-oriented programming (OOP), objects are the central building blocks. These objects represent real-world entities, such as a car, a student, or a bank account. But before we can use these objects, we need to create them. This is where constructors come in. In Java, constructors are special methods that are responsible for initializing the state of an object when it is created. They play a crucial role in ensuring that objects are properly set up and ready to be used.
Understanding Constructors: The Foundation of Object Creation
Imagine you're building a house. Before you can move in, you need to lay the foundation. Similarly, before you can use a Java object, you need to initialize its data members (also known as fields or attributes). Constructors are like the foundation of an object – they establish the initial state of the object's data members.
A constructor is a method with the same name as the class. It has no return type (not even
void
) and is invoked automatically whenever a new object is created using the
new
keyword.
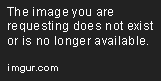
Key Characteristics of Constructors
-
Constructor name is the same as the class name: This allows the compiler to identify them as special methods for object initialization.
-
No return type: Constructors do not return any value, including
void
. -
Automatically invoked during object creation: When you use the
new
keyword to create an object, the constructor of that class is automatically called.Types of Constructors
Java offers different types of constructors to cater to various object creation scenarios. - Default Constructor
If you don't explicitly define any constructors in your class, Java provides a default constructor. This constructor has no parameters and initializes data members to their default values (e.g., 0 for integers,
false
for booleans,null
for references).
-
No return type: Constructors do not return any value, including
class Employee {
String name;
int age;
// Default constructor (implicitly created)
public Employee() {
// Data members initialized to default values
}
}
- Parameterized Constructors
You can create constructors that accept parameters. This allows you to initialize data members with specific values during object creation.
class Employee {
String name;
int age;
// Parameterized constructor
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
}
- Copy Constructor
A copy constructor creates a new object that is a copy of an existing object. It takes an object of the same class as a parameter.
class Employee {
String name;
int age;
// Copy constructor
public Employee(Employee other) {
this.name = other.name;
this.age = other.age;
}
}
Example: Creating an Employee Object
Let's see how to use constructors to create an
Employee
object.
class Employee {
String name;
int age;
// Parameterized constructor
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
// Method to display employee details
public void displayDetails() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
// Creating an Employee object using the parameterized constructor
Employee employee1 = new Employee("Alice", 30);
// Displaying employee details
employee1.displayDetails();
}
}
Output:
Name: Alice
Age: 30
In this example:
- We define an
Employee
class with data membersname
andage
. - The parameterized constructor takes the employee's name and age as input and initializes the corresponding data members.
- In the
main
method, we create anEmployee
object namedemployee1
using thenew
keyword and passing the name and age as arguments to the constructor. - The constructor initializes the data members of the
employee1
object. - We then call the
displayDetails()
method to print the employee's information.Constructor Chaining
Constructor chaining allows you to call one constructor from another constructor within the same class. This is useful for reusing code and ensuring consistent initialization logic. It uses the
this()
keyword to call another constructor within the same class.
class Employee {
String name;
int age;
String department;
// Constructor 1: Initialize name and age
public Employee(String name, int age) {
this.name = name;
this.age = age;
this.department = "General"; // Default department
}
// Constructor 2: Initialize name, age, and department
public Employee(String name, int age, String department) {
this(name, age); // Call constructor 1 to initialize name and age
this.department = department;
}
}
In this example:
- Constructor 2 calls constructor 1 using
this(name, age)
. This ensures that name and age are initialized by constructor 1, and then constructor 2 sets the department.Best Practices for Constructors
- Initialization of all data members: Ensure that all data members of the class are initialized within the constructor.
- Avoid complex logic: Keep the logic inside the constructor as simple as possible to maintain readability and avoid potential errors.
- Use defensive programming: Validate input parameters to prevent unexpected behavior, like handling null or invalid values.
- Consider copy constructors: If your class has mutable data members, consider using a copy constructor to prevent unintended modifications to the original object.
-
Use constructor chaining wisely: Constructor chaining can simplify the process of initializing objects with different sets of values, but ensure it doesn't lead to excessive nesting or complex logic.
Conclusion
Constructors are essential in Java object-oriented programming. They act as the starting point for creating objects and setting their initial state. By understanding the different types of constructors, constructor chaining, and best practices, you can effectively build robust and well-initialized objects. This foundation is crucial for creating complex and efficient Java applications.