<!DOCTYPE html>
Server-Side Rendering (SSR) in Next.js
<br> body {<br> font-family: sans-serif;<br> line-height: 1.6;<br> margin: 0;<br> padding: 20px;<br> }<br> h1, h2, h3 {<br> margin-top: 2em;<br> }<br> pre {<br> background-color: #f5f5f5;<br> padding: 10px;<br> border-radius: 5px;<br> overflow-x: auto;<br> }<br> code {<br> font-family: monospace;<br> }<br> img {<br> max-width: 100%;<br> height: auto;<br> }<br>
Server-Side Rendering (SSR) in Next.js
Introduction
In the world of web development, the way a web page is rendered has a significant impact on its performance, user experience, and SEO. Server-Side Rendering (SSR) is a technique that addresses some of the shortcomings of client-side rendering, making it a popular choice for building high-performing and SEO-friendly web applications.
Next.js, a popular React framework, provides built-in support for SSR, simplifying the process and enabling developers to easily create fast and robust applications. This article will guide you through the concepts, techniques, and practical examples of implementing SSR in Next.js.
Why Server-Side Rendering Matters
Client-side rendering, where JavaScript runs in the browser to generate the webpage, has become the standard for modern web applications. However, it comes with certain drawbacks:
-
Slow initial load time:
The browser needs to download the entire JavaScript bundle before rendering, leading to a delay in displaying content. -
Poor SEO:
Search engines typically struggle to crawl and index client-side rendered pages as they rely on JavaScript to generate content. -
Difficult to share links:
Sharing a link to a specific state of a client-side rendered page may not work as expected.
SSR tackles these issues by rendering the HTML on the server before sending it to the browser. This has several advantages:
-
Faster initial load times:
Users see content quicker as the HTML is already served from the server. -
Improved SEO:
Search engines can easily crawl and index the pre-rendered content. -
Better link sharing:
Links to specific states can be shared and accessed directly.
Server-Side Rendering with Next.js
Next.js simplifies SSR by providing a seamless and intuitive approach. By default, Next.js automatically renders all pages on the server, making it incredibly straightforward to create SSR-powered applications.
- Creating a Next.js Project
Let's start by creating a basic Next.js project. You can use the following command in your terminal:
npx create-next-app@latest my-ssr-app
This command will create a new directory named "my-ssr-app" with the necessary files and dependencies. You can then navigate into the directory:
cd my-ssr-app
getStaticProps
and getServerSideProps
Next.js provides two essential functions for SSR: getStaticProps
and getServerSideProps
. These functions are used to fetch data on the server before rendering the page.
getStaticProps
is used for static generation, where data is fetched at build time. This is ideal for data that is rarely updated, such as blog posts or product listings. The data is then baked into the HTML at build time, making it extremely fast for subsequent requests.
getServerSideProps
is used for dynamic generation, where data is fetched on each request. This is suitable for dynamic content like user profiles, real-time updates, or data that changes frequently.
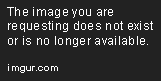
Let's illustrate the use of these functions with an example. We'll create a simple page that displays a list of products fetched from an API.
pages/products.js
import { getStaticProps } from 'next';
const Products = ({ products }) => {
return (
Products
-
{products.map(product => (
-
{product.name}
${product.price}
))}
);
};
export const getStaticProps = async () => {
const res = await fetch('https://fakestoreapi.com/products');
const data = await res.json();
return {
props: {
products: data,
},
};
};
export default Products;
In this code, we define a component called "Products" that renders a list of products. The getStaticProps
function fetches data from the "fakestoreapi.com" API and passes it as props to the component.
- Running the Application
You can now run your Next.js application to see the results:
npm run dev
This will start a development server, and you can access the application at http://localhost:3000. If you navigate to http://localhost:3000/products, you should see the list of products fetched from the API and rendered on the server.
Advanced SSR Techniques in Next.js
Next.js offers several advanced features that enhance SSR capabilities, enabling more complex scenarios and optimizing performance.
You can also fetch data directly within a component using the useSWR
hook from the swr
library. This allows you to dynamically update data without refreshing the entire page.
import useSWR from 'swr';
const Products = () => {
const { data: products, error } = useSWR('https://fakestoreapi.com/products', fetch);
if (error) return
Failed to load products;if (!products) return Loading products...;
return (
Products
-
{products.map(product => (
-
{product.name}
${product.price}
))}
);
};
export default Products;
The useSWR
hook handles caching and data fetching, making it efficient and easy to manage dynamic content updates.
- Static Site Generation (SSG)
For highly static content, Next.js offers Static Site Generation (SSG). This pre-renders the entire site at build time, resulting in the fastest possible load times and optimal SEO. You can utilize SSG by using the getStaticProps
function and ensuring your data is static or pre-fetched during the build process.
Example: Blog Post Page
import { getStaticProps } from 'next';const BlogPost = ({ post }) => {
return (
{post.title}
{post.content}
);
};export const getStaticProps = async ({ params }) => {
const res = await fetch(https://api.example.com/posts/${params.slug}
);
const data = await res.json();
return {
props: {
post: data,
},
};
};export default BlogPost;
By fetching data at build time, Next.js will pre-render the blog post page, making it extremely fast and efficient.
- Incremental Static Regeneration (ISR)
ISR provides a balance between SSG and SSR. It pre-renders content at build time, but can be updated dynamically based on a defined schedule or when data changes. This allows you to have fast initial page loads with the flexibility of updating content without full server re-renders.
Example: Product Page
import { getStaticProps, getStaticPaths } from 'next';const Product = ({ product }) => {
return (
{product.name}
${product.price}
);
};export const getStaticPaths = async () => {
const res = await fetch('https://fakestoreapi.com/products');
const data = await res.json();
const paths = data.map(product => ({ params: { id: product.id.toString() } }));
return { paths, fallback: true };
};export const getStaticProps = async ({ params }) => {
const res = await fetch(https://fakestoreapi.com/products/${params.id}
);
const data = await res.json();
return {
props: {
product: data,
},
revalidate: 60, // Revalidate every 60 seconds
};
};export default Product;
In this example, getStaticPaths
generates static paths for each product, and getStaticProps
fetches data and revalidates the page every 60 seconds, ensuring content stays updated.
- API Routes
Next.js allows you to define API routes that are handled directly by the server, enabling you to create serverless functions. This is useful for tasks like data validation, authentication, or accessing external APIs.
Example: Authentication API
pages/api/auth.js
import { NextApiRequest, NextApiResponse } from 'next';export default async function handler(req: NextApiRequest, res: NextApiResponse) {
if (req.method === 'POST') {
// Handle authentication logic
const { username, password } = req.body;
// ... authenticate user
res.status(200).json({ message: 'Authentication successful' });
} else {
res.status(405).json({ message: 'Method not allowed' });
}
}
You can access this API route from your frontend using the fetch
API or other methods.
Conclusion
Server-side rendering in Next.js offers a compelling way to build high-performing, SEO-friendly web applications. By leveraging the built-in features of Next.js, you can easily implement SSR, SSG, and ISR, tailoring the rendering strategy to meet your specific application needs.
Remember to consider the following best practices:
-
Optimize data fetching:
Use efficient methods to retrieve data and minimize API calls. -
Prioritize user experience:
Ensure a seamless user experience by providing loading states and handling errors gracefully. -
Leverage caching:
Utilize caching strategies to optimize data retrieval and reduce server load. -
Monitor performance:
Regularly monitor your application's performance to identify and address potential bottlenecks.
With a solid understanding of SSR principles and the capabilities of Next.js, you can create engaging and performant web applications that deliver exceptional user experiences.