<!DOCTYPE html>
useState() in React: A Comprehensive Guide
<br> body {<br> font-family: sans-serif;<br> line-height: 1.6;<br> margin: 0;<br> padding: 20px;<br> }<br> h1, h2, h3 {<br> margin-top: 2em;<br> }<br> code {<br> background-color: #f5f5f5;<br> padding: 5px;<br> border-radius: 3px;<br> }<br> img {<br> max-width: 100%;<br> display: block;<br> margin: 20px auto;<br> }<br>
useState() in React: A Comprehensive Guide
In the world of React, managing state is fundamental. State refers to any data that your application needs to keep track of and that can change over time. This data could be anything from the current value of a form input to the list of items in a shopping cart.
React provides a built-in Hook called
useState()
to make state management a breeze. This powerful tool allows you to easily track and update data within your functional components, simplifying your component logic and promoting code reusability.
What is useState()?
useState()
is a React Hook that lets you add state to a functional component. It's a simple yet powerful tool that helps you build dynamic and interactive user interfaces.
Here's how it works:
-
Initialization:
You call
with an initial value for your state. This value is what your component will start with.
useState()
-
Returns a pair:
returns an array with two elements:
useState()
-
The current state:
This is the value of your state variable. -
A function to update the state:
This function allows you to change the value of your state variable.
-
Why use useState()?
Before the introduction of Hooks, state management in functional components was cumbersome and required the use of higher-order components or other workaround solutions.
useState()
changed the game by bringing the simplicity and power of state management directly into functional components.
Here are some key benefits of using
useState()
:
-
Simplicity:
provides a straightforward way to manage state within your functional components, making your code cleaner and more readable.
useState()
-
Code Reusability:
You can easily extract state management logic from your components into reusable custom Hooks, promoting modularity and maintainability. -
Better Performance:
By directly working with state within components,
allows React to optimize re-rendering, ensuring your applications run efficiently.
useState()
Using useState() in Practice
Let's illustrate how
useState()
works with a practical example: A simple counter component.
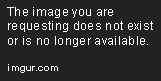
Here's the code for this counter component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // Initialize state to 0
const handleClick = () => {
setCount(count + 1); // Update state
};
return (
<div>
<h1>
Count: {count}
</h1>
<button onclick="{handleClick}">
Increment
</button>
</div>
);
}
export default Counter;
In this example, we do the following:
-
We import
from the
useState
library.
react
-
We call
to initialize a state variable named
useState(0)
with an initial value of 0.
count
-
returns an array:
useState()
-
The first element,
, holds the current value of the counter.
count
-
The second element,
, is a function that we can use to update the value of
setCount
.
count
-
The first element,
-
Inside the
function, we call
handleClick
to increment the counter. This triggers a re-render of the component, updating the displayed count.
setCount(count + 1)
Understanding Re-rendering
One of the key things to understand about
useState()
is that updating state causes your component to re-render. React efficiently compares the new state with the old state and only re-renders parts of your application that have changed, minimizing unnecessary re-renders and optimizing performance.
State Update Mechanisms
While you can update state directly like in our counter example,
useState
offers more powerful ways to update state:
-
Functional updates:
This allows you to update state based on the previous state. This is particularly useful when you need to perform calculations or derive the new state based on the old state. -
Asynchronous updates:
You can update state with asynchronous operations like fetching data from an API. React handles this gracefully, ensuring that state updates occur at the right time.
Functional Updates
Consider a scenario where you need to update the state of a counter but ensure that the new value is always an even number:
function EvenCounter() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(prevCount => prevCount + 2); // Functional update
};
return (
<div>
<h1>
Count: {count}
</h1>
<button onclick="{handleClick}">
Increment (Even)
</button>
</div>
);
}
In this example, we use a function to update
count
. This function takes the previous state (
prevCount
) as an argument and returns the new state (
prevCount + 2
), ensuring the counter remains even.
Asynchronous Updates
Let's say you want to fetch data from an API and update the state of your component with the fetched data:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
const [isLoading, setIsLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
setIsLoading(true); // Show loading indicator
const response = await fetch('https://api.example.com/data');
const data = await response.json();
setData(data); // Update state with fetched data
} catch (error) {
setError(error);
} finally {
setIsLoading(false); // Hide loading indicator
}
};
fetchData();
}, []);
if (isLoading) {
return
<p>
Loading...
</p>
;
}
if (error) {
return
<p>
Error: {error.message}
</p>
;
}
return (
<div>
{data && ( // Render data only if it's available
<ul>
{data.map((item) => (
<li key="{item.id}">
{item.name}
</li>
))}
</ul>
)}
</div>
);
}
export default FetchData;
In this example, we use the
useEffect
Hook to fetch data from the API when the component mounts. Inside the
useEffect
function:
-
We call an asynchronous function
to handle the API call.
fetchData
-
We update the
state to
isLoading
before fetching data to display a loading indicator.
true
-
We update the
state with the fetched data after the response is successfully received.
data
-
If there's an error, we update the
state.
error
-
Finally, we set
back to
isLoading
to hide the loading indicator.
false
Combining useState() with Other Hooks
useState()
often works hand-in-hand with other React Hooks to create powerful and complex components. Let's explore some common combinations:
useEffect
We already saw how to use
useEffect
to fetch data. Here are some other use cases for
useEffect
combined with
useState
:
-
Setting up subscriptions:
You can use
to subscribe to events and update your state based on those events. For example, you could subscribe to a WebSocket connection and update a state variable when you receive new messages.
useEffect
-
Performing side effects:
allows you to run code that has side effects. This might include things like setting up timers, accessing the DOM, or performing calculations that don't directly affect your component's render.
useEffect
useRef
useRef
gives you a way to create a reference to a DOM element or any other value within your component. It's useful for scenarios like directly manipulating DOM elements or storing values that don't trigger re-renders.
import React, { useState, useRef } from 'react';
function FocusInput() {
const [inputValue, setInputValue] = useState('');
const inputRef = useRef(null); // Create a reference
const handleClick = () => {
inputRef.current.focus(); // Focus on the input element
};
return (
<div>
<input =="" onchange="{(e)" type="text" value="{inputValue}"/>
setInputValue(e.target.value)}
ref={inputRef} // Assign the reference
/>
<button onclick="{handleClick}">
Focus Input
</button>
</div>
);
}
export default FocusInput;
In this example, we create a
ref
using
useRef(null)
. Then, we assign the reference to our input element using the
ref
prop. We can later access this
ref
to programmatically focus on the input element.
Best Practices
Following these best practices can help you write clean and efficient code when using
useState()
:
-
Use functional updates:
Always use functional updates whenever possible. This helps ensure that your state updates are based on the previous state, preventing issues with race conditions and unexpected behavior. -
Avoid unnecessary re-renders:
Only update state when it's absolutely necessary. If you only need to update a small part of your component's UI, consider using techniques like memoization or updating only the relevant state variables. This will improve your application's performance. -
Keep your state minimal:
Don't try to manage all of your application's state in a single component. Break your application into smaller components and manage state at the appropriate level. This will improve code organization and maintainability. -
Use state management libraries for complex apps:
For more complex applications with intricate state management, consider using a state management library like Redux, MobX, or Zustand. These libraries provide a more structured approach to handling state, particularly when your application scales.
Conclusion
useState()
is an essential tool in the React developer's toolkit. It enables you to manage state effectively within your functional components, leading to cleaner code, better performance, and more dynamic user interfaces. By understanding the concepts behind
useState()
, its various update mechanisms, and its integration with other Hooks, you can build robust and responsive React applications. Remember to follow best practices to ensure clean, maintainable, and performant code.